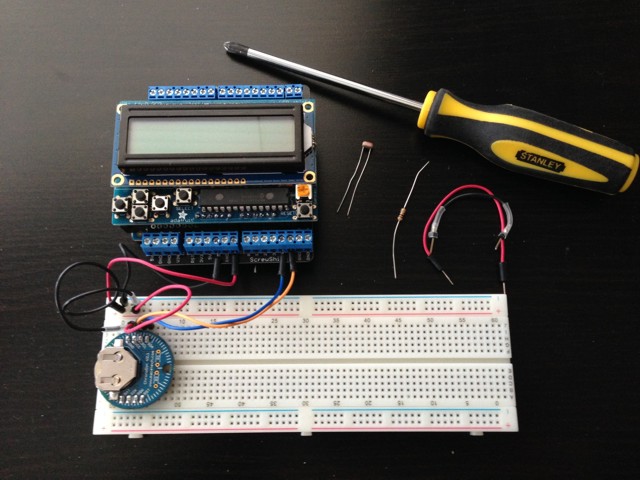
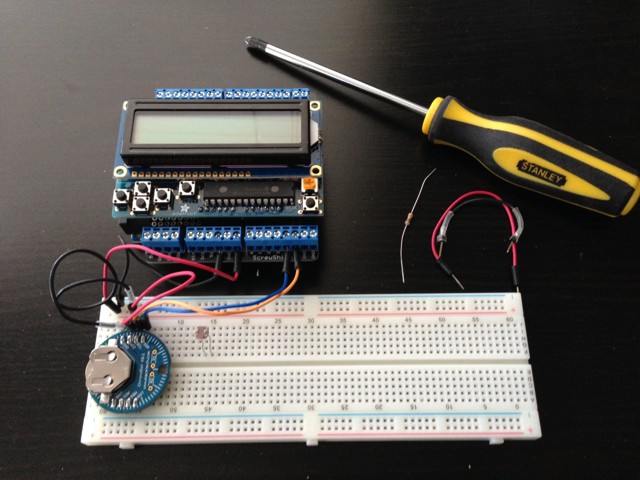
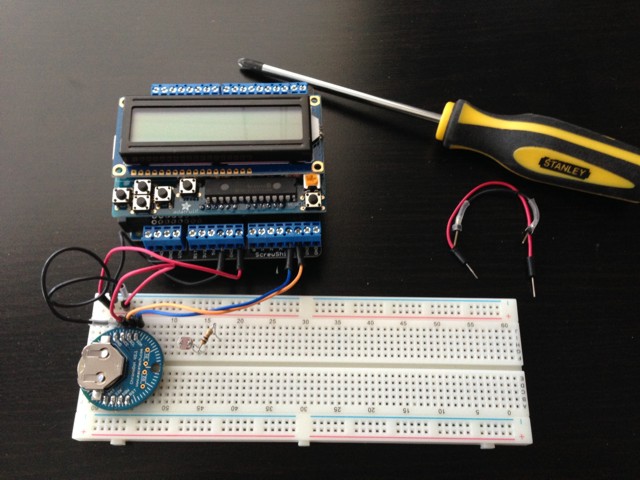
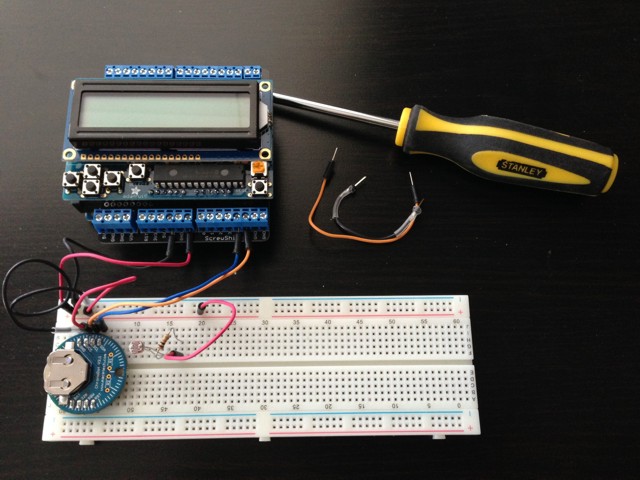
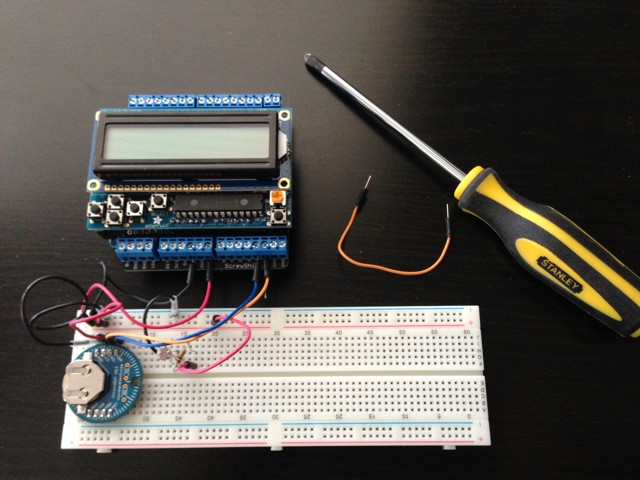
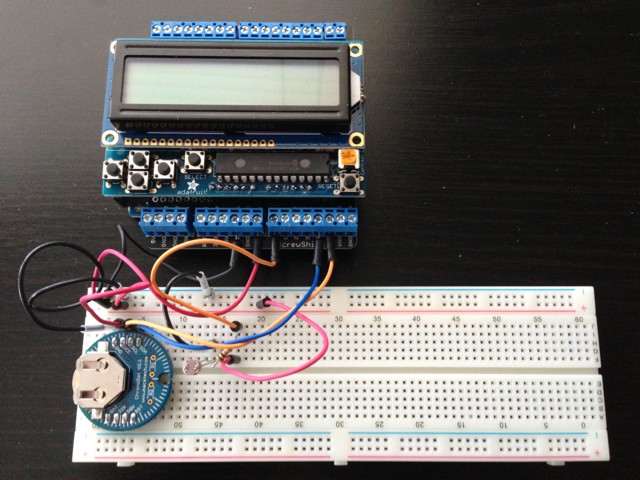
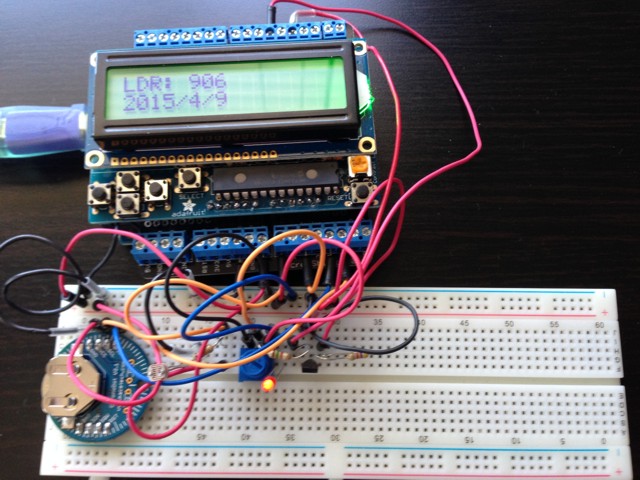
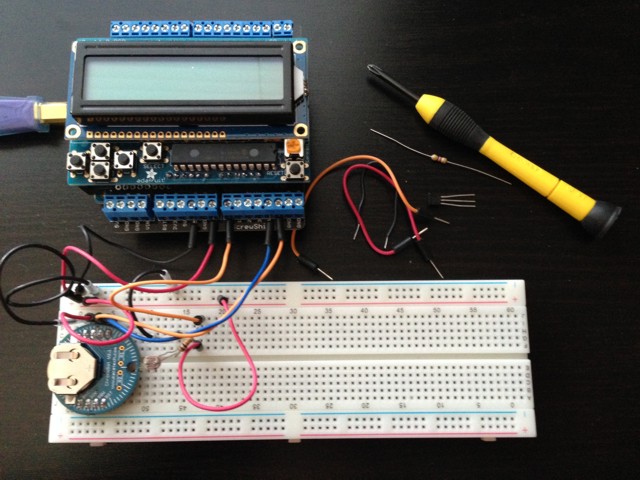
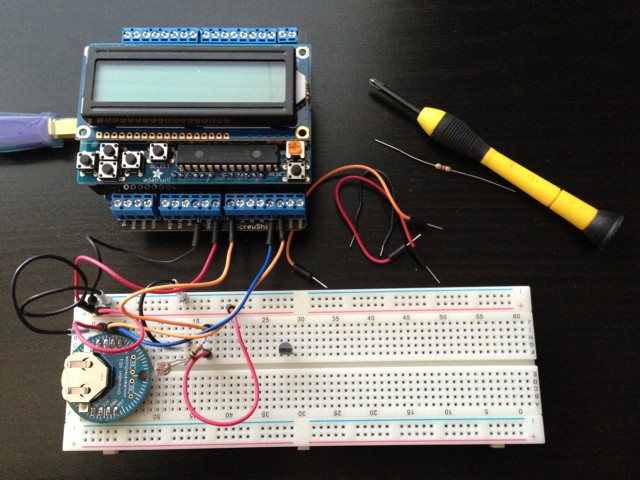
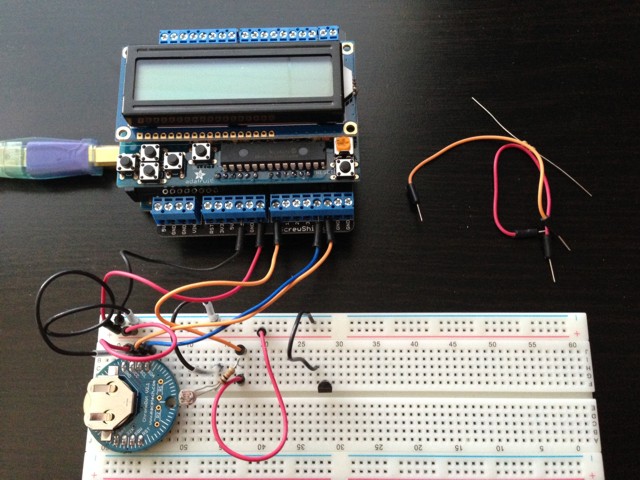
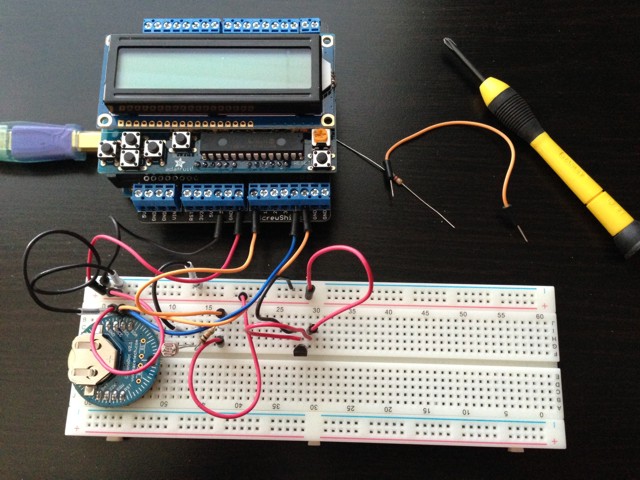
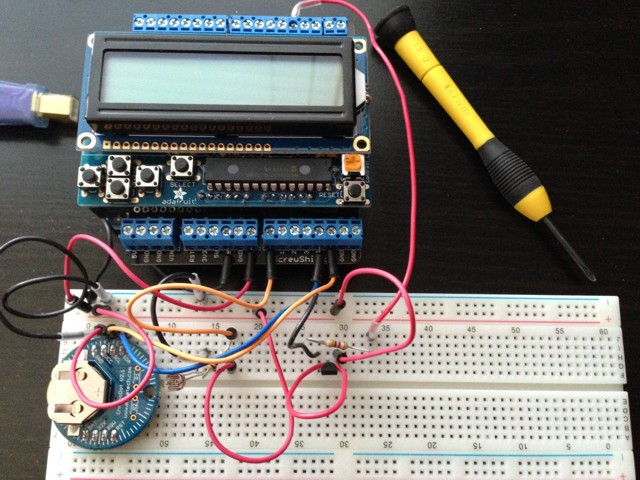
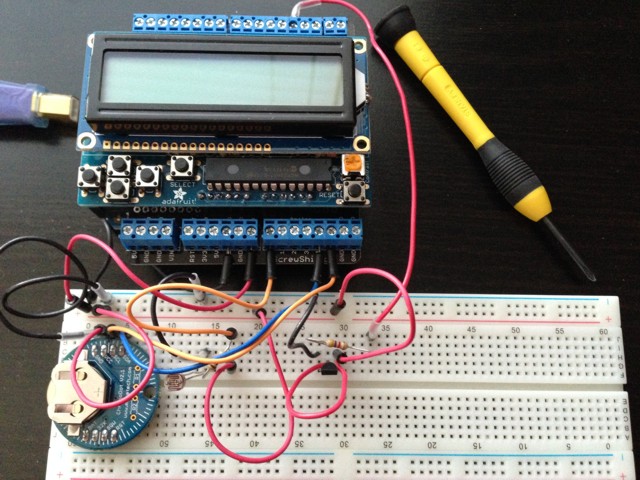
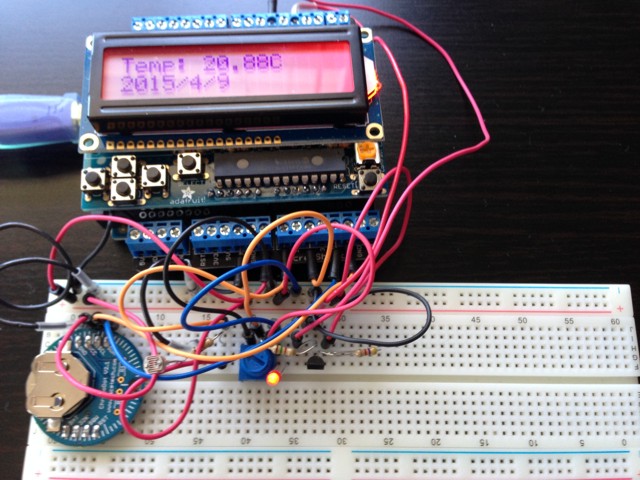
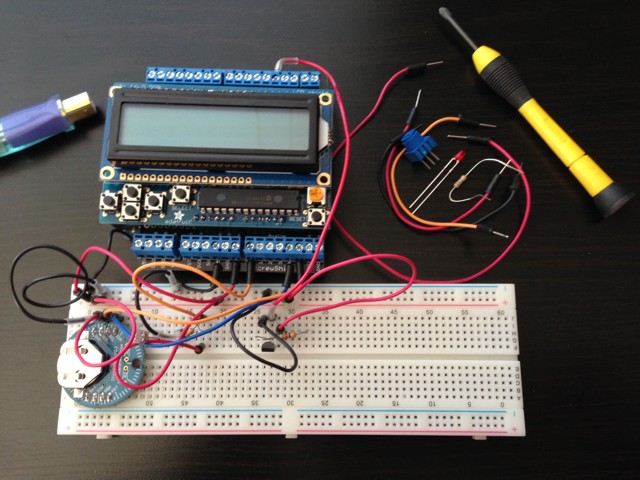
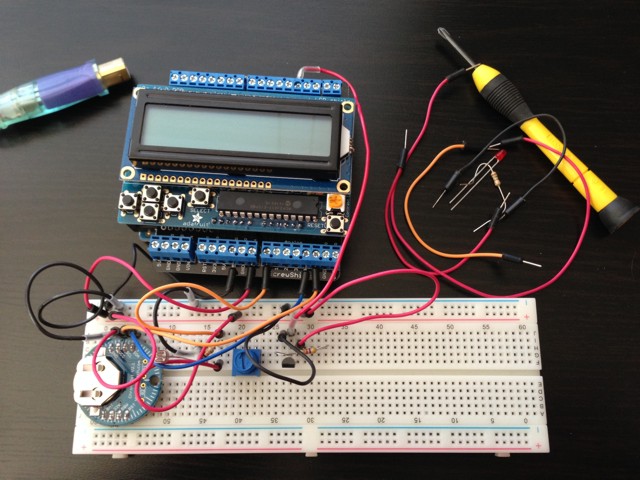
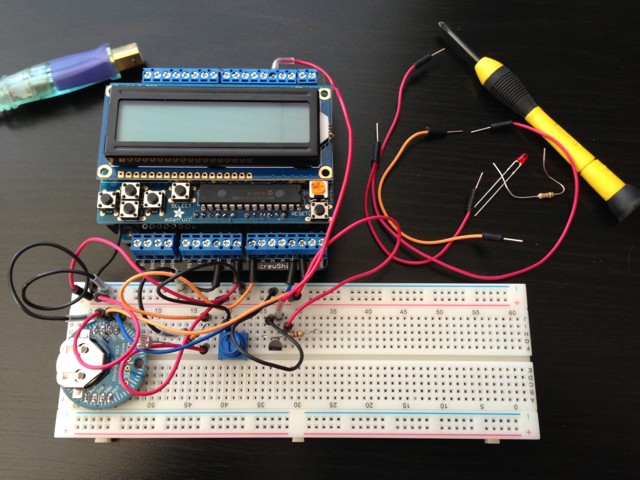
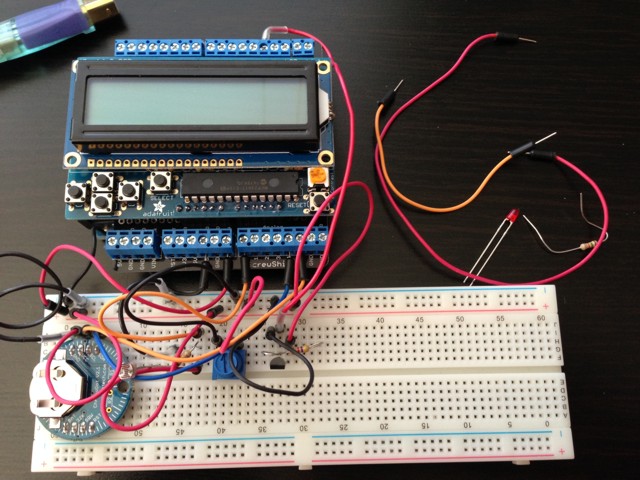
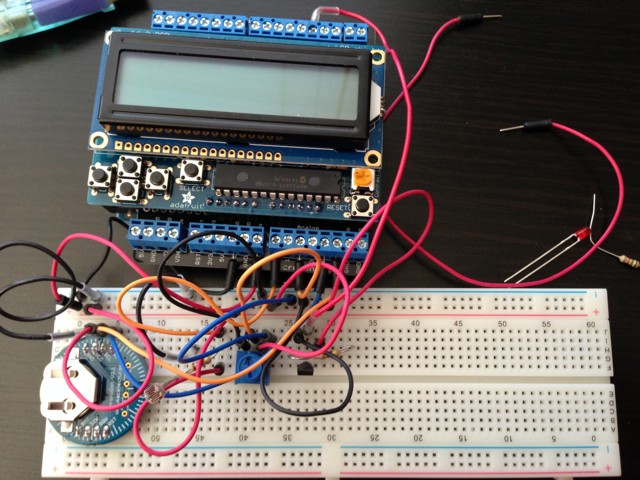
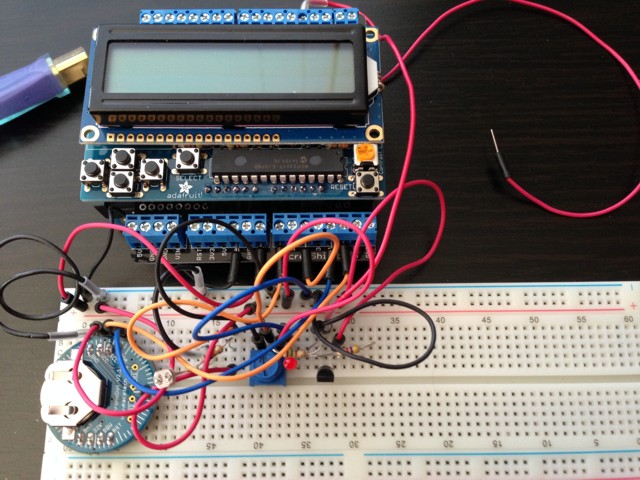
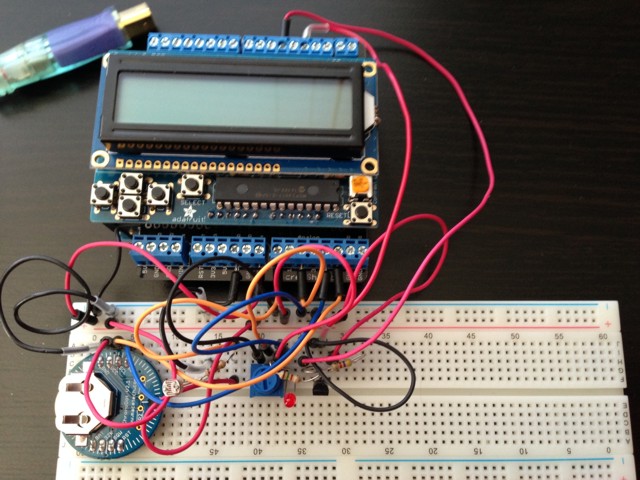
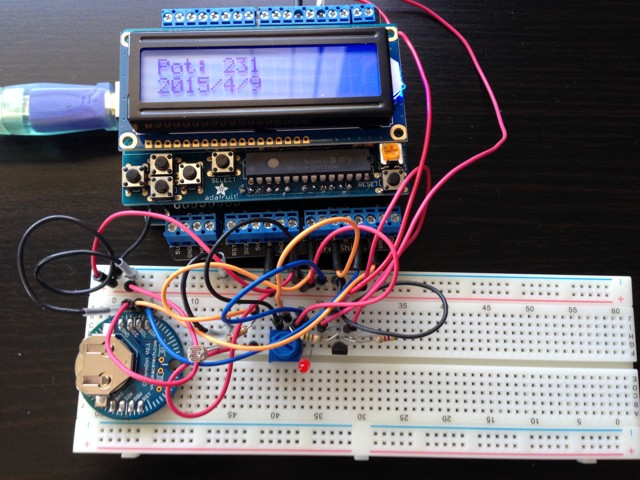
1 X Mini Photocell
1 X Resistor 10k Ohm
1 X One Wire Digital Temperature Sensor – DS18B20
1 X Resistor 4.7k Ohm
1 X Trimpot 10K with Knob
1 X Resistor 1.65k Ohm
1 X 3MM Low Current Red LED
14 X Jumper Wires Premium 3″ M/M
1 X Project #3 – LED Shield – Mk3
LCDShieldMk4.3.ino
// ***** Don Luc *****
// Software Version Information
// 4.3
// include the library code:
#include <Wire.h>
#include <Adafruit_MCP23017.h>
#include <Adafruit_RGBLCDShield.h>
#include <SPI.h>
#include <RTClib.h>
#include <RTC_DS3231.h>
#include <OneWire.h>
RTC_DS3231 RTC;
#define SQW_FREQ DS3231_SQW_FREQ_1024 //0b00001000 1024Hz
Adafruit_RGBLCDShield RGBLCDShield = Adafruit_RGBLCDShield();
// These #defines make it easy to set the backlight color
#define OFF 0x0
#define RED 0x1
#define YELLOW 0x3
#define GREEN 0x2
#define TEAL 0x6
#define BLUE 0x4
#define VIOLET 0x5
#define WHITE 0x7
// Chorno
boolean isChorno = false;
char datastr[100];
// LDR (light dependent resistor)
int LDR_Pin = A0;
String LDR = "";
// Temperature chip i/o
int DS18S20_Pin = 2; //DS18S20 Signal pin on digital 2
OneWire ds(DS18S20_Pin); // on digital pin 2
String tempZ = "";
// Potentiometer
int potPin = A2; // select the input pin for the potentiometer
int ledPin = 4; // select the pin for the LED
boolean isVal = false;
int potPot = 0;
String cap = "";
void loop() {
// timeChrono();
timeChrono();
uint8_t momentaryButton = RGBLCDShield.readButtons();
if ( momentaryButton ) {
RGBLCDShield.clear();
RGBLCDShield.setCursor(0,0);
if ( momentaryButton & BUTTON_UP ) {
timeLDR();
RGBLCDShield.print( LDR );
RGBLCDShield.setBacklight(GREEN);
}
if ( momentaryButton & BUTTON_DOWN ) {
temperatu();
RGBLCDShield.print( tempZ );
RGBLCDShield.setBacklight(RED);
}
if ( momentaryButton & BUTTON_LEFT ) {
getPotentio();
RGBLCDShield.print( cap );
RGBLCDShield.setBacklight(BLUE);
}
if ( momentaryButton & BUTTON_RIGHT ) {
RGBLCDShield.print("YELLOW - RIGHT");
RGBLCDShield.setBacklight(YELLOW);
}
if ( momentaryButton & BUTTON_SELECT ) {
RGBLCDShield.print("OFF");
RGBLCDShield.setBacklight(OFF);
}
}
delay(3000);
}
setup.ino
void setup() {
// set up the LCD's number of columns and rows:
RGBLCDShield.begin(16, 2);
RGBLCDShield.print("Don Luc!!!");
RGBLCDShield.setBacklight(VIOLET);
// ChronoDot
setupChrono();
// Pot
pinMode(ledPin, OUTPUT);
}
ChronoDot.ino
void setupChrono() {
RTC.begin();
DateTime now = RTC.now();
DateTime compiled = DateTime(__DATE__, __TIME__);
RTC.getControlRegisterData( datastr[0] );
}
void timeChrono() {
DateTime now = RTC.now();
DateTime isNow (now.unixtime() + 5572 * 86400L + 26980);
// set the cursor to column 0, line 1
RGBLCDShield.setCursor(0, 1);
if ( isChorno == false )
{
isChorno = true;
RGBLCDShield.print(isNow.year(), DEC);
RGBLCDShield.print('/');
RGBLCDShield.print(isNow.month(), DEC);
RGBLCDShield.print('/');
RGBLCDShield.print(isNow.day(), DEC);
RGBLCDShield.print(' ');
RGBLCDShield.print(' ');
}
else if ( isChorno == true )
{
isChorno = false;
RGBLCDShield.print(isNow.hour(), DEC);
RGBLCDShield.print(':');
RGBLCDShield.print(isNow.minute(), DEC);
RGBLCDShield.print(':');
RGBLCDShield.print(isNow.second(), DEC);
RGBLCDShield.print(' ');
RGBLCDShield.print(' ');
}
}
getLDR.ino
void timeLDR() {
// LDR
int LDRReading = analogRead(LDR_Pin);
LDR = "LDR: ";
LDR.concat(LDRReading);
}
getPot.ino
void getPotentio() {
if ( isVal == false )
{
isVal = true;
digitalWrite(ledPin, HIGH); // turn the ledPin on
}
else if ( isVal == true )
{
isVal = false;
digitalWrite(ledPin, LOW); // turn the ledPin off
}
potPot = analogRead(potPin); // read the value from the sensor
cap = "Pot: ";
cap.concat(potPot);
}
getTemperature.ino
float getTemp() {
//returns the temperature from one DS18S20 in DEG Celsius
byte data[12];
byte addr[8];
if ( !ds.search(addr)) {
//no more sensors on chain, reset search
ds.reset_search();
return -1001;
}
if ( OneWire::crc8( addr, 7) != addr[7]) {
return -1002;
}
if ( addr[0] != 0x10 && addr[0] != 0x28) {
return -1003;
}
ds.reset();
ds.select(addr);
ds.write(0x44,1); // start conversion, with parasite power on at the end
byte present = ds.reset();
ds.select(addr);
ds.write(0xBE); // Read Scratchpad
for (int i = 0; i < 9; i++) { // we need 9 bytes
data[i] = ds.read();
}
ds.reset_search();
byte MSB = data[1];
byte LSB = data[0];
float tempRead = ((MSB << 8) | LSB); //using two's compliment
float TemperatureSum = tempRead / 16;
return TemperatureSum;
}
void temperatu(){
float temperature = getTemp();
tempZ = "Temp: ";
tempZ.concat(temperature);
tempZ.concat("C");
}
Don Luc